06.09
Most games nowadays avoid hardcoding behavior in the main program code. It makes the development process a lot easier by allowing people with less programming experience than the core engine developers to contribute by writing scripts which defines how conversations happen in the game, how menus work, how cinematic scenes go, etc. Scripts are usually written in a higher level language than the game engine, as they require less performance and must be portable when the game needs to run on different platforms. Easy, common script languages like Lua or Python are often used (for example, CCP uses Python to describe the EVE Online client GUI, and Microsoft uses Lua in Freelancer to describe cinematics), but some companies like to create their own language and their own interpreter to do this job.
I'm a fan of Namco's "Tales of" RPG games. I was given a Wii last december as a gift, and bought "Tales of Symphonia: Dawn of the New World". As a true hacker interested in game development, after finishing the game, I started investigating how this game actually works behind the scenes. After writing software to read the encrypted Wii disc of the game, I analyzed the data contained on the DVD, trying to find what was each file and how the game engine made all of this into a game.
For those who don't know, the Tales RPG often have a system to display optional conversation between the game protagonists, which are not directly tied to the game scenario but sometimes give more precision on characters or on some story points from before the game. Some examples on Youtube. These are known as "skits" and are displayed as the characters 2D portraits talking and being animated on the screen. I thought this was a good starting point to try to understand how the game works, and thus tried to find the files related to these skits on the disc. What I'm exposing in this article is work that took me about 3 to 4 months and ended at the start of April.
First, using Dolphin, the best (and probably the only) Wii emulator, I logged all the disc files being opened using the "file monitor" feature of this emulator. When opening the first skit in the game, the emulator output was:
W[FileMon]: 31 kB Chat/FC01_001.bin
W[FileMon]: 4 kB Chat/e/FC01_001.bin
I[FileMon]: 248 kB Sound/stream/FC01_001.brstm
It was easy enough to understand that Chat/ probably contained graphics elements as well as a description of the character picture animation, and Sound/ contained the voices of the characters. Let's not talk about the sound data (maybe in a next article, but they are basically 4-bit ADPCM encoded sound files) and concentrate ourselves on the Chat/ files.
Both of the .bin files are actually Microsoft Cabinet archives (.cab) which can easily be extracted with cabextract or any program using libmspack. Note that one is at the root of the Chat/ directory, while one is in the Chat/e/ directory. Actually, here are all the FC01_001.bin files:
./e/FC01_001.bin
./g/FC01_001.bin
./f/FC01_001.bin
./s/FC01_001.bin
./i/FC01_001.bin
./FC01_001.bin
If you've played the game, it's easy enough to understand what are these directories. The european version of the game I'm working on have been released in five languages: english, german, french, spanish and italian. Looking at the size of the files (31K for Chat/FC01_001.bin and 4.1K for the language specific ones), we can assume that the non language specific one contains only images while the others contains the subtitles for example. Let's extract these .cab files!
In the non language specific one:
-rw-r--r-- 1 119K Aug 28 2008 ar.dat
In the english one:
-rw-r--r-- 1 33K Aug 15 2009 FC01_001.so
Both of these files seem to be in an unknown format. ar.dat does not even have a magic number in its header, but FC01_001.so starts with "TSS\0" which nobody seems to have heard of on the internet. There are a few strings in the .so file: subtitles, as expected, but also things like restTime (%f) or TCP balloon(%d). Not looking too good so far! That's when static analysis of the files start to show its limits, and it's time to run the Dolphin debugger to find what is actually accessing the .so file when it is loaded in memory. First, I paused the code execution while it was displaying a skit and dumped the contents of the RAM. By locating the "TSS\0" string, I found out that the .so file was loaded at offset 0x816D8394 when executing the skit. I proceeded to modify the PowerPC interpreter in the Dolphin emulator to dump the CPU state in JSON at each memory read or write in the zone containing the .so file, and ran the skit once more to get a full dump of what code is actually accessing our file.
First, there seems to be some copying going on : the same instruction is writing data in the zone from top to bottom, starting at 0x816E0393 down to 0x816D8394. Classic memcpy, nothing to see here. After that, the four first bytes are read by an instruction at 0x80071E0C. Let's fire IDA to see what this is about:
.text2:80071E0C lwz %r3, 0(%r21)
.text2:80071E10 addis %r0, %r3, -0x5453
.text2:80071E14 cmplwi %r0, 0x5300
.text2:80071E18 beq loc_80071E40
If you are not familiar with PowerPC assembly, what this does line by line is loading a word (32 bytes) at the address contained in r21, then add -0x54530000 to it, and compare it to 0x5300. In other words, it compares the first four bytes of the file to 0x54535300, which is "TSS\0", the four characters code which describe the file type. The rest of the code in this function is very large, let's not waste time and go to the next memory access to find an interesting one.
Some fields in the header seems to be read : offsets 0x4, 0xC, 0x14, 0x18, 0x1C, 0x8, 0x10, and then a lot of repeated accesses from code at address 0x80091DBC on always different offsets (0x7D40, 0x7D48, then 0x742C, etc.). Let's look at the code control flow there:
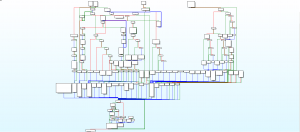
An experienced eye may directly recognize a switch statement here: loads of code without a visible predecessor, all leading to the same exit. I'd even go further and assume that is the main loop of a script interpreter. That would make of the .so file a Script Object file, containing compiled bytecode. Sounds consistent. If we look more precisely at the code 0x80091DBC which is reading the bytecode in memory, we can see that it is used to choose where to jump in the switch. In other words, it is the dispatch code of the bytecode interpretation loop, reading an opcode and dispatching it to handling code. From there, we can get nice informations: if it loads the opcode from memory then we can find where is the "current instruction" pointer, aka. PC (program counter). Let's look for that! This is the interesting part of the code:
lwz %r0, 0xC(%r15)
lwz %r4, 4(%r15)
mulli %r0, %r0, 0x1420
add %r5, %r15, %r0
lwz %r3, 0x142C(%r5)
lwzx %r17, %r4, %r3 # Read opcode
The last instruction loads a word indexed from memory. This means it loads the word at address r4 + r3. Looking at the memory dump we did with Dolphin, we can see the value of those two registers: 'r3':'00007D40', 'r4':'816D83B4'. So r4 is the address of the bytecode file (0x816D8394) + 0x20, which we can assume to be the address of the first opcode in the file, after the bytecode header. r3 is the PC, which varies while we are executing the code. They are both stored in some sort of interpreter state, whose address is stored in r15. In this state is the code base address, at offset 0x4 (second instruction of the listing), and some sort of table index at offset 0xC (loaded in the first instruction of the listing, then multiplied by a constant, and used as an index). This index is used to find the current PC. From that, we can assume that there can be multiple PCs stored at the same time, depending on the value of r15[0xC]. Hm...
Let's increase the usefulness of our memory dump by also dumping memory accesses to the whole interpreter state. After dumping all of that, we have log files containing 188MB of JSON with the CPU state at each access. In the next part of this article, I'll explain how to make this dump useful and readable by filtering it a little bit, and we'll start analyzing the 20 instructions of this bytecode 🙂
For impatient people, the final result of this reverse engineering work is a completely rewritten version of the bytecode interpreter, which can be found on my Bitbucket : cscript-interpreter. This is able to execute skit scripts quite well, even if there is still a bit of reverse engineering to do on the syscalls part.
did u know u can also download an iso dump from the net and explore the files using dolphin, looking at assembly code is completely unecessary.
Looking at the files is not the problem, it’s understanding what is in these files and writing the bytecode interpreter to run on a PC.
I used wiiodfs to access the contents of the ISO dump.
@wasteoftime: I assume you haven’t read the article completely? Your comment reads moronic.
I love these walkthroughs, keep writing more.
I didn’t even know there was a Wii emulator that worked, let alone one as powerful as Dolphin. Is it easy to load wii games on it?
It actually works really well. There are obviously still a few issues but they are mostly minor and games are often completely playable. Dolphin was originally a GC emulator which evolved into a Wii emulator (the CPU/GPU/DSP architecture is almost the same on those two consoles) so most of the code is quite mature and have been tested a lot.
I think they are currently working towards a 3.0 release soon, that may be a good time to try it (but the SVN versions are often really stable).
Thanks for sharing. Interesting and informative
[…] Part 1 of this article talked about how I found the bytecode files and the interpreter assembly code. Let's go further and try to understand how these bytecode files are structured. […]
This was awesome, many thanks
Can’t wait to see an analysis of the instructions cause I’m really interested in this sort of engines.
What revision of Dolphin did you use for the “file monitor” feature? It doesn’t seem to work on any of my versions. 🙁
Looks like the file monitor only displays messages with “info” verbosity. I didn’t catch this because I actually patched Dolphin to be able to show info and debug level log messages using a release build (by default you need to use a debug build to be able to see info and debug logs).
Apply this patch, recompile Dolphin, and set the verbosity to “info” in the log configuration window:
Index: Source/Core/Common/Src/Log.h
===================================================================
— Source/Core/Common/Src/Log.h (revision 7590)
+++ Source/Core/Common/Src/Log.h (working copy)
@@ -100,7 +100,7 @@
#define MAX_LOGLEVEL DEBUG_LEVEL
#else
#ifndef MAX_LOGLEVEL
-#define MAX_LOGLEVEL WARNING_LEVEL
+#define MAX_LOGLEVEL DEBUG_LEVEL
#endif // loglevel
#endif // logging
Could you upload a debugbuild or a fast debugbuild of Dolphin please?
[…] from none other than Delroth’s Twitter account. Delroth is a legend in the emulator world, and his blog on reverse engineering offers plenty of insights on the hobby. It only took him three months to write the Eri HaKawai […]
[…] none other than Delroth’s Twitter account. Delroth is a legend in the emulator world, and his blog on reverse engineering offers plenty of insights on the hobby. It only took him three months to write the Eri HaKawai […]